Multiple addresses
The extra
object
extra
objectThe extra
object can pass multiple wallets to the widget so the customer can switch between cryptocurrencies during the purchase without manually entering a different wallet address.
When passing wallet addresses in the extra
object, please keep in mind that:
- Users can always edit the pre-filled addresses.
- Addresses will remain pre-filled if the user switches between cryptocurrencies.
- If you provide addresses for the main cryptocurrency in both the
address
option and theextra
object, the one inaddress
will be pre-filled. - The
extra
object only functions with our SDK.
Please ensure you pass the correct values in commodities
and wallets.
You can find commodity
values in Supported Coins and Blockchains.
The wallet object structure
You can define an array of default wallets that will be pre-filled when the user switches between cryptocurrencies.
Property | Type | Description |
---|---|---|
name | string | Example: ETH , please refer to the list of supported currencies. |
network | string | Example: ethereum , please refer to list of supported currencies. |
address | string | The user's wallet address. Non-valid addresses will be ignored. |
Extra
object structure:
extra: {
item_info: Object,
wallets: Array,
}
Examples
- You can provide the required crypto asset and the user's address if it's a single-wallet address. The widget will load with the provided address:
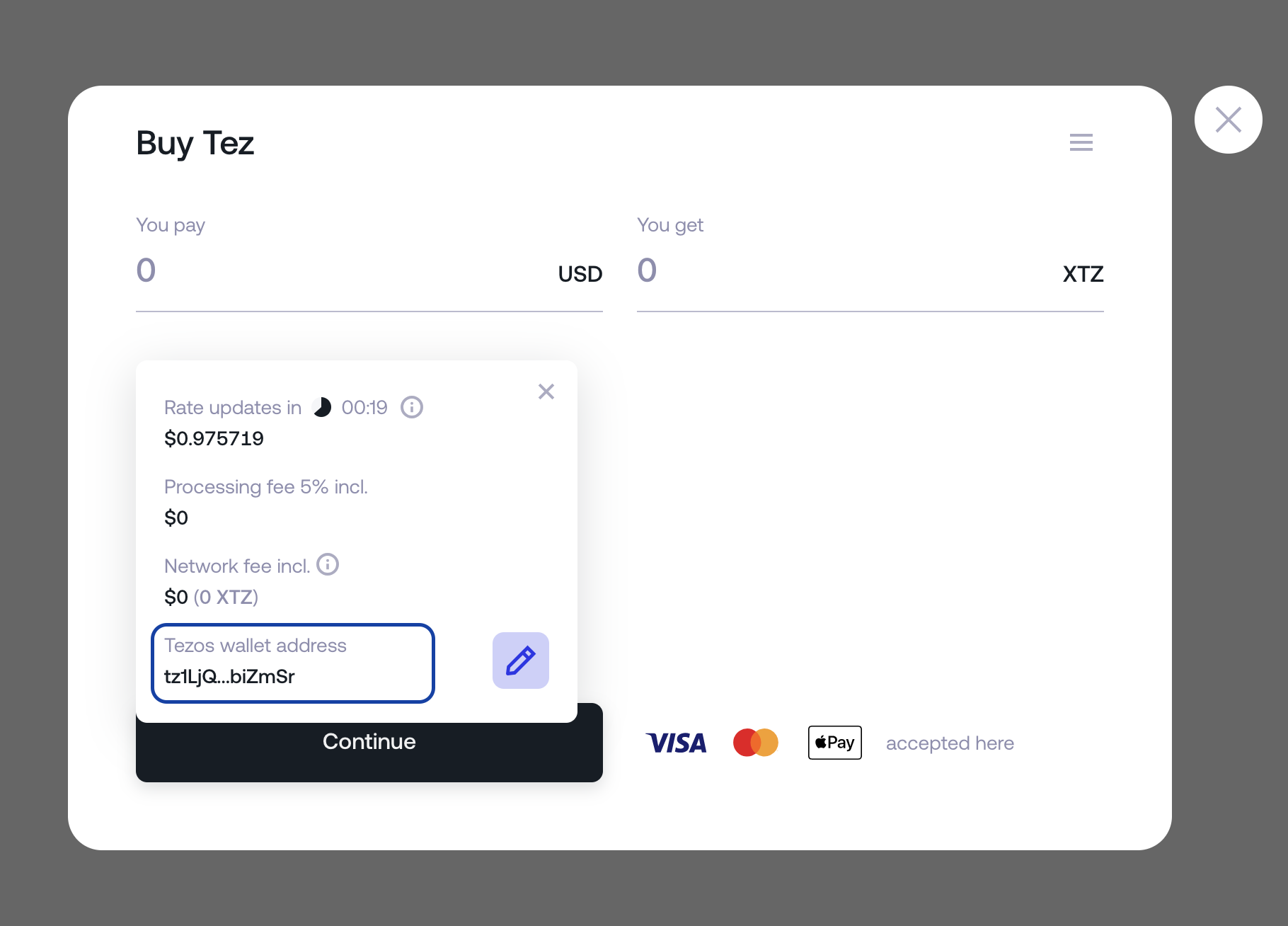
Example of providing a single wallet address:
const wertWidget = new WertWidget({
// ... other properties
commodity: "XTZ",
network: "tezos",
address: "tz1LjQKVfHCCCYm8DDYKPzL5w4nCtobiZmSr",
commodities: JSON.stringify([
{
commodity: "XTZ",
network: "tezos",
},
]),
});
- You can display all commodities in the widget but provide addresses only for some:
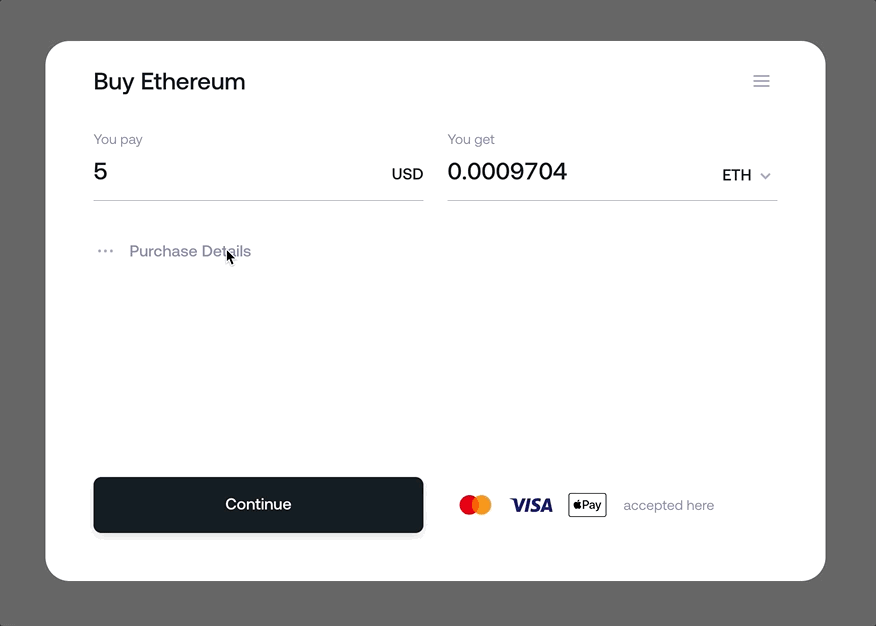
Example of providing addresses for some commodities:
In the example below three addresses will be pre-filled, the user can manually enter other addresses.
const options: Options = {
// ... other properties
currency: 'USD',
commodity: 'ETH',
network: 'ethereum',
extra: {
wallets: [
{
name: "ETH", // case-ignored
network: "ethereum", // case-ignored
address: "0x0E976df9bb3ac63F7802ca843C9d121aE2Ef22ee",
},
{
name: "XTZ", // case-ignored
network: "tezos", // case-ignored
address: "tz1LjQKVfHCCCYm8DDYKPzL5w4nCtobiZmSr",
},
{
name: "MATIC", // case-ignored
network: "polygon", // case-ignored
address: "0x0E976df9bb3ac63F7802ca843C9d121aE2Ef22ee",
},
],
},
};
- You can hide certain commodities in the widget and display only specific ones and their corresponding addresses. The widget will load with the selected commodities and pre-filled addresses:
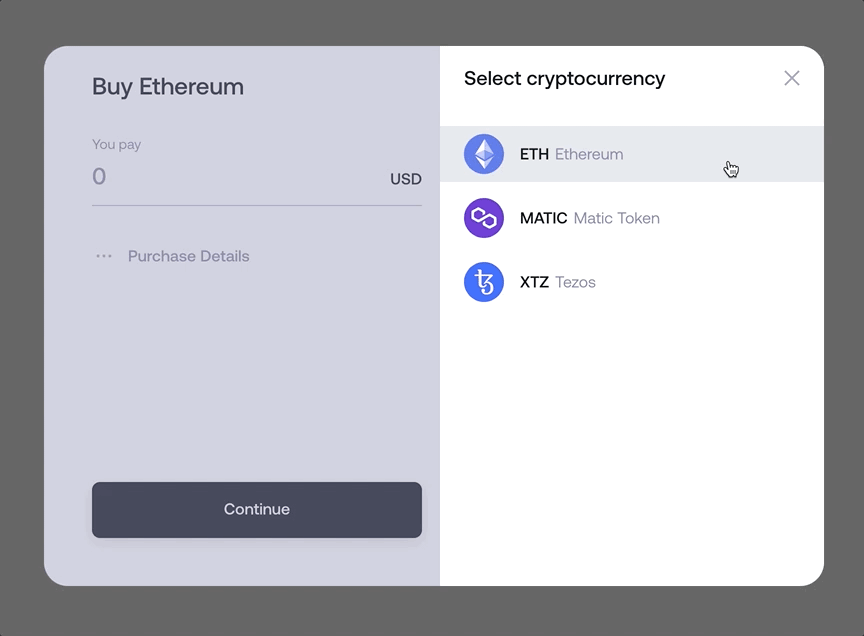
Example of hiding some commodities and providing addresses for the selected ones:
const wertWidget = new WertWidget({
// ... other properties
commodities: JSON.stringify([
{
commodity: "ETH",
network: "ethereum",
},
{
commodity: "XTZ",
network: "tezos",
},
{
commodity: 'MATIC',
network: 'polygon',
},
]),
extra: {
wallets: [
{
name: "ETH", // case-ignored
network: "ethereum", // case-ignored
address: "0x0E976df9bb3ac63F7802ca843C9d121aE2Ef22ee",
},
{
name: "XTZ", // case-ignored
network: "tezos", // case-ignored
address: "tz1LjQKVfHCCCYm8DDYKPzL5w4nCtobiZmSr",
},
{
name: "MATIC", // case-ignored
network: "polygon", // case-ignored
address: "0x0E976df9bb3ac63F7802ca843C9d121aE2Ef22ee",
},
],
},
});
Updated about 1 year ago
Documentation related to this page